Using Const in C++
So what is const? Const means constant... Why should you use const? You should use const when you don't need to edit the value etc.
I'm by no means an expert, but if I made a typo feel free to reach out.
class Example
{
public:
Example() {}
// const on the end means you "promise" that the method won't edit any values in the class Example (can get around this by marking a variable mutable)
int GetA() const { return m_A; }
// If you're going to return a pointer you can add const before and after the * just like below (ugly)
const int* const GetB() const { return m_B; }
private:
int m_A;
int* m_B;
};
int main()
{
// const before a * means you can’t modify the contents of the pointer
// const after a * means you can’t modify the pointer
// const before and after adds both above
int VALUE = 1337;
int* a = new int(1);
const int* b = new int(50);
int* const c = new int(100);
const int* const d = new int(8000);
// You can also do int const* instead of const int* which means the same thing as they're before the *
std::cout << "A: " << *a << std::endl;
std::cout << "B: " << *b << std::endl;
std::cout << "C: " << *c << std::endl;
std::cout << "D: " << *d << std::endl;
*a = 2; // We can set the value
// *b = 10; // We can't set the value of b since const is before the *
b = &VALUE; // We can set the pointer
*c = 25; // We can set the value
// c = &a; // We can't set the pointer to something else
// We can't do anything to d as we specify const before and after the * (there are ways around stuff like this though)
// *d = 80;
// d = &CONST_VALUE;
std::cout << "A: " << *a << std::endl;
std::cout << "B: " << *b << std::endl;
std::cout << "C: " << *c << std::endl;
std::cout << "D: " << *d << std::endl;
std::cin.get();
}
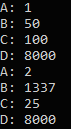
Also, generally when passing a struct or a user-defined type you should pass by const reference like so unless you want to copy or even edit that value.
void TestMethod(const Example& ex)
{
}
until next time